| Posted By
 Superglob on 2021-05-21 12:08:15
| Software Sprites
Hi There!
What's the most 'popular' way to do software sprites on the c16/plus4? I'm assuming it's pre-shifted frames, either single or multi-color, and then just poking tiles into memory if you don't care about the background. How many games actually do a logical-OR with the background? Any hints / tips people want to share?
Assuming self-modifying code would make the most sense for speed?
-SG
|
|
Posted By
 Csabo on 2021-05-21 18:30:21
| Re: Software Sprites
These questions are a big too vague, but I'll try to cover them.
Hmm, most popular? I don't think there's a consensus on this. I'd say the approach has always been that each coder implements whatever is needed for their game/demo.
How many games - this would be too difficult to count, but there are certainly "some" 
Self-modifying code being the fastest - again, this really depends on what you're trying to do.
One thing I can recommend is take a look at RoePipi's page, at the bottom he has a list of his favourite games which doubles as a showcase of "sprites done right". To mention two at random; check out Bongo and Rescue from Zylon - both really well done but very different beasts.
|
|
Posted By
 Luca on 2021-05-21 19:33:10
 | Re: Software Sprites
If your question is about "objects which don't interact with both themselves and a background" they're similar to the Blitter OBjects from Amiga, so we should better call'em "Bobs", and, as Csabo has correctly pointed out, their coding depends on your conditions (chars or bitmap, for example). Btw I remember that it it was Csabo who taught me how to code some bobs to enrich my part in Crackers' Demo 4...
Mad has shared his sprite routines used for Pets Rescue, if it could be of some help.
|
|
Posted By
 Superglob on 2021-05-21 22:51:57
| Re: Software Sprites
Thanks both!
Yeah, it's maybe a bit vague as each game has different needs. For games like MOTR, a lot of it can just be character based with just the main character doing a logical OR against the background. For more advanced ones though, it becomes more complicated and you'll probably have to do some sort of bucketing,.
Anyone know what mad shared his a routines?
Much appreciate you guys giving constructive criticism There's precious little info on the c16 / plus 4 in terms IMO compared to the vic and 64. Then again, maybe I'm just not looking hard enough. Pointers like these help a lot!
-SG
|
|
Posted By
 Luca on 2021-05-22 02:38:53
 | Re: Software Sprites
You can download the sprites'source code of the game directly from the game's related page Pets Rescue, there's a .zip file with sources and demos.
Basically, the software sprites routine has a core routine made of a sequence of LDA AND ORA STA cycles, and analysing that a bit closer, there are some duties to perform before, and some better choices made of illegal opcodes and few other smart decisions about that  In general, you have to: - restore background from the previous frame:
sprow23 ldy #$52 ; restore background lda s2char9 sta (origin2),y dey lda s2char8 sta (origin2),y dey lda s2char7 sta (origin2),y sprow22 ldy #$2a lda s2char6 ... - choose next anomation frame and point to all the needed chars; - look for the new location where to print the new chars and store the untouched background chars; - do the magic using that same background, where to overlap the portion of the sprites chosen by the needed Y-shifting:
... seekch1 lda charset,x and (sp_and),y ora (sp_ora),y sta spriteset,x ...
Certainly, this is just one, of the different ways you can perform a software sprite (in chars in this case), reductions optimization and improvements can differ.
This is one frame of the 4-frame stars moving over and behind a 5x5 scroller which I coded for Smells Like Happy Birthday demo, in this worksheet you can locate three sets: the original graphics, the AND mask, the ORA with transparency: the first has evident use, the second is used to have the right "hole" into the background portion, the third uses the background color ($00) in order to ORA the rest of the graphics without involving the whole char box and differs from the previous one in a way that you can have in any condition a "contour" of the sprite coloured like background color, which is the "mask" of the sprite if you like to have one (I didn't, in that demo).
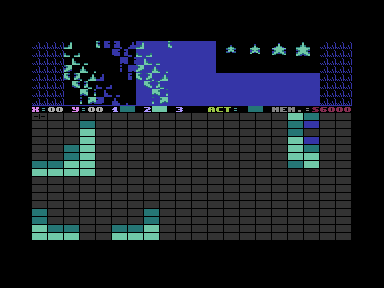
|
|
Posted By
 Mad on 2021-05-23 21:52:11
| Re: Software Sprites
There is some technique called automasking.. Where you don't need a mask. Actually color 0,1,2 are opaque there and color 3 is transparent. But that is a bit more advanced, but it helped in our games to have up to 200 and more sprite frames in ram whilst doing all the rest. With masks it would have been only 100 sprite frames.. And shifting is not a good idea, too if you want much memory.. With masks and preshifts the number of sprite frames would be 1/8 of what is actually possible memory wise (25 sprite frames instead of 200 sprite frames)..
This is the code for applying automasking, where ora automask,x is the interessting part, this table is a bit more complicated to calculate but once you got it everything is easy and you don't need masks anymore whilst keeping the same speed. lax (ZP_SPRITEDATA),y ; sprite and (ZP_BACKGROUNDDATA),y ; background and sprite ora automask,x sta (ZP_SPRITEDATA),y ; sprite
For non preshifted sprites you may use tables to shift your sprites in realtime.. the shifting code would look like ldx leftcolumnbyte,y lda shifttable1,x (for instance this tables shifts in this case by 2 bit to the left) ldx rightcolumnbyte,y ora shifttable2,x (in this case this table shifts by 6 bit to the right (thus shifts in 2 bits from above))
you would have to exchange the shifttables by self modifying code. this is of course optimizable if you do several bytes at once in one row. (ldx rightcolumnbyte,y is ldx leftcolumnbyte,y of the next byte, so no need to load it again)
Selfmodifying code is the key.. And unrolling the innerloops of course! If you target the C16 most probably other people may help you, I think many of these games just shift the sprites when needed, but others know more about that.
Xeo3 uses some sort of sprite caching which is a plus if the same spritegraphic is shown on consecutive frames. So you only shift the graphics once and use it again if needed.
Whats also good is to never save the background of the sprites, instead repaint the graphics from the tilemap behind the sprites.. If you would save the background you would need to draw them one after another but clear them in reverse order, and most of the times this approach is probably slower..
If you do charmode it's good if the sprites only use the 3 frozen colors ($ff15,$ff16,$ff17) so you avoid colorclashes. In bitmap mode sprite painting is a lot of another field, there other rules apply.. But the easy way is of course also possible in bitmap mode, but it gets very slow if you don't use tricks there.
If I remember right Pets Rescues Routine can do 6-8 24x32 sprites in 50Hz.. Xeo3 probably even more.. So actually many big sprites are no problem for this machine.
I don't know anything about what people used in the past like all the other pro people here, most of them know more about "popular techniques". Maybe other people will help you more..
Happy coding!
|
|
Posted By
 Superglob on 2021-05-24 17:36:19
| Re: Software Sprites
Thanks (again) for all the detailed explanations! Much appreciated!
|
|
| |
Copyright © Plus/4 World Team, 2001-2024 |