Posted By
 orac81 on 2025-03-15 14:19:35
| SCONNEX
Ok, so heres an improved algorithm version of CONNEX, in C:
Download: https://github.com/orac81/miniapples/raw/refs/heads/main/sconnex-latest.zip
https://github.com/orac81/miniapples/blob/main/README.md#sdl-connex
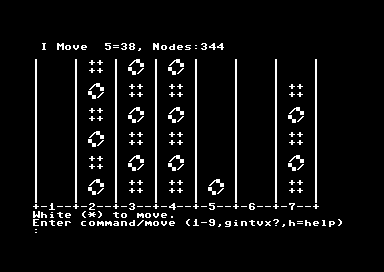
(c16/+4 console vbcc version)
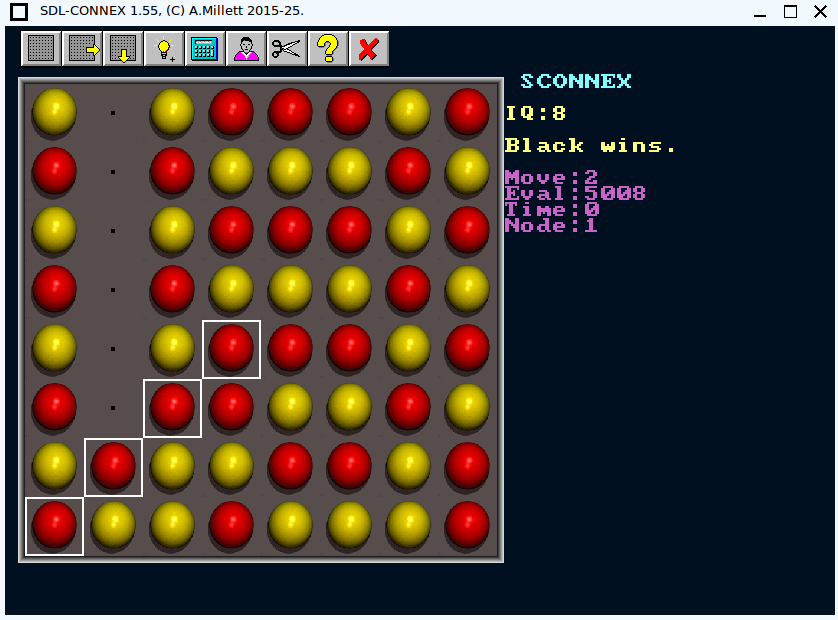 (SDL version)
SCONNEX is a version of the famous 4-in-a-line game, written to work on almost any computer. It can compile to work as an SDL app (screen shot above) or as a C99 terminal program. The compiled executable is only 7k for the 6502.
Now I have experimented compiling C99 sconnex with vbcc (see the cbm dir in zip), and it outperforms the cc65 by about 40%, so you can play a game on level iq5+ (5 ply) at a few seconds a move on a 1mhz 6502. As the game progresses deeper searches can be used. It needs time regulation/iterative deepening to work best, but thats not yet on this "simple" version of the program.
The program uses the "classic" recursive AlphaBeta search pruning algorithm which looks like this:
int AlphaBeta(int depth, int alpha, int beta) { if (depth == 0) return Evaluate(); GenerateLegalMoves(); while (MovesLeft()) { MakeNextMove(); val = -AlphaBeta (depth - 1, -beta, -alpha); UnmakeMove(); if (val >= beta) return beta; if (val > alpha) alpha = val; } return alpha; }
(I am sure most coders have seen this before, but I have seen programs just using brute force minmax, so its worth mentioning.)
I think an asm version should speed the code up by a factor of 5 or so, and there is plenty of room for improvement, the search is quite simple. Still this version plays quite well, and could be built for almost any machine, the console version only needs putchar and gets supplied (no printf!).
To build the code, change these defines in sconnex.c
#define IS_SDL 0 #define IS_C99 1
then simply compile:
cc sconnex.c -Os
I was trying to make a vbcc version for the vic20 or c16/+4, but the is no lib yet in vbcc. A vic20 version would be simple, nearly the same as the c64, the basic rom is near identical, just moved from $a000 to $c000. Also basic start for 8k+ machines would be $1201 rather than $0801. an option for starting at $2001 would be useful so that $1000-1fff can be used for hires gfx.
To get unix time() in vbcc, a simple hack is to make long var peeking the jiffy clock, then divide by 60. (I didnt leave that in this version.)
A generic CBM target might be useful, just using std kernal calls, and a user supplied code start, zp area, jiffy clock ptr etc.
Anyway, thoughts welcome.
|
|
Posted By
 Csabo on 2025-03-15 15:52:23
| Re: SCONNEX
Hey, now that you're a registered member, would you like to take a crack and add this game to the site? You have the rights In fact every member does, my biggest goal is to encourage everyone to add/edit everything as needed.
Let me know, we can talk about the details in PMs if you like (otherwise we'll add it).
|
|
Posted By
 orac81 on 2025-03-18 14:22:20
| Re: SCONNEX
Thanks, If you want you can add it. This version is just a console/terminal version in C, with just simple scrolling ascii graphics. I was thinking of adding the petscii gfx from the old basic version. also the author of Vbcc has added an experimental plus4 option which is about twice the speed of cc65. That may be of wider interest to others here. If you look at the source, you will see that I used a type XINT which is just byte, vbcc performs well with byte integer variables.
vbcc/c16:iq7,n,g :mv4,84sec,16489nod (196 node/sec) cc65/c16:iq7,n,g :mv4,176sec,16489nod (94 node/sec)
Here is a c16 version compiled with vbcc: http://forum.6502.org/download/file.php?id=21743
|
|
|